OpenZeppelin's Ownable: A User Guide
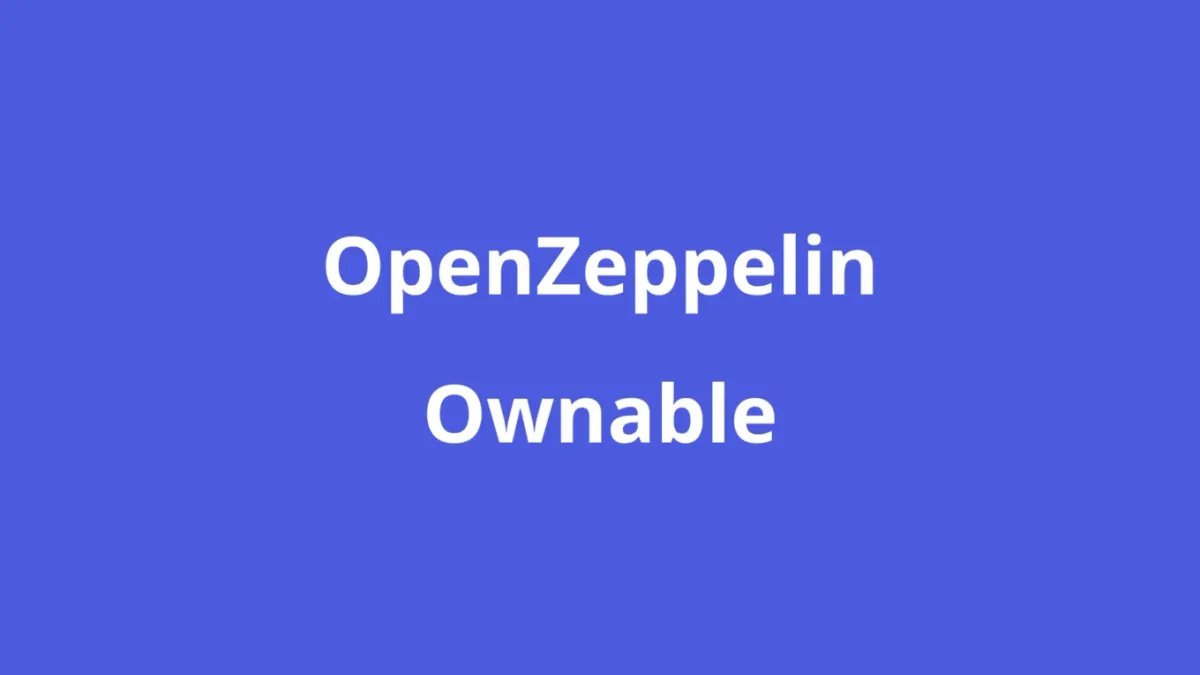
In this post, we'll dive into one of the libraries from OpenZeppelin, known as Ownable. We'll briefly outline the benefits of using this library:
- Control over functions and more through ownership
- Enhanced security
- Simplified implementation of ownership transfer
Let's delve into the details!
What is Ownable?
OpenZeppelin's Ownable allows you to manage the ownership of a contract. Unlike web2, smart contracts are accessible to anyone. However, there are functions that you might want only the owner (the creator) to use. Ownable helps you safely handle ownership in such cases.
It can be used in various scenarios, like withdrawing tokens, minting or burning NFTs. Without proper control, your NFTs could be burned maliciously, or your tokens could be stolen, making control essential.
Inside Ownable
Let's take a look at what's inside:
// SPDX-License-Identifier: MIT
// OpenZeppelin Contracts (last updated v5.0.0) (access/Ownable.sol)
pragma solidity ^0.8.20;
import {Context} from "../utils/Context.sol";
abstract contract Ownable is Context {
address private _owner;
error OwnableUnauthorizedAccount(address account);
error OwnableInvalidOwner(address owner);
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
constructor(address initialOwner) {
if (initialOwner == address(0)) {
revert OwnableInvalidOwner(address(0));
}
_transferOwnership(initialOwner);
}
modifier onlyOwner() {
_checkOwner();
_;
}
function owner() public view virtual returns (address) {
return _owner;
}
function _checkOwner() internal view virtual {
if (owner() != _msgSender()) {
revert OwnableUnauthorizedAccount(_msgSender());
}
}
function renounceOwnership() public virtual onlyOwner {
_transferOwnership(address(0));
}
function transferOwnership(address newOwner) public virtual onlyOwner {
if (newOwner == address(0)) {
revert OwnableInvalidOwner(address(0));
}
_transferOwnership(newOwner);
}
function _transferOwnership(address newOwner) internal virtual {
address oldOwner = _owner;
_owner = newOwner;
emit OwnershipTransferred(oldOwner, newOwner);
}
}
It's quite simple. Let's look at the functions:
modifier
modifier onlyOwner() {
_checkOwner();
_;
}
function _checkOwner() internal view virtual {
if (owner() != _msgSender()) {
revert OwnableUnauthorizedAccount(_msgSender());
}
}
This modifier allows the owner to pass through successfully, and reverts if the caller is not the owner.
owner()
function owner() public view virtual returns (address) {
return _owner;
}
This function allows you to call the owner's address in your functions.
transferOwnership
function transferOwnership(address newOwner) public virtual onlyOwner {
if (newOwner == address(0)) {
revert OwnableInvalidOwner(address(0));
}
_transferOwnership(newOwner);
}
function _transferOwnership(address newOwner) internal virtual {
address oldOwner = _owner;
_owner = newOwner;
emit OwnershipTransferred(oldOwner, newOwner);
}
As the name suggests, this function transfers ownership to another address. This allows you to transfer ownership to another address without any issues.
renounceOwnership()
function renounceOwnership() public virtual onlyOwner {
_transferOwnership(address(0));
}
Use this function with caution! It completely renounces ownership, leaving the contract without an owner and disabling any functions marked with onlyOwner
.
How to Use Ownable
You might be wondering how to use it. It's quite simple:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.21;
import "@openzeppelin/contracts/access/Ownable.sol";
contract MyContract is Ownable {
uint256 public value;
constructor(address initialOwner) Ownable(initialOwner) {
value = 0;
}
function increment() external {
value++;
}
function decrement() external {
require(value > 0, "Value cannot be negative");
value--;
}
function setValue(uint256 _value) external onlyOwner {
value = _value;
}
}
Inherit Ownable in your contract like this (don't forget to import it):
import "@openzeppelin/contracts/access/Ownable.sol";
contract MyContract is Ownable {
Then, pass the address you want to have ownership to the constructor:
constructor(address initialOwner) Ownable(initialOwner) {}
That's it! You can now use other functions and controls from Ownable to make your smart contract safer.
Implementing Your Own Ownable
If you don't need the full functionality of Ownable and just want simple ownership control, you can implement it like this:
contract MyContract2 {
address private _owner;
constructor(address initialOwner) {
_owner = initialOwner;
}
modifier onlyOwner() {
require(_owner == msg.sender, "Ownable: caller is not the owner");
_;
}
}
It's surprisingly simple and easy. However, if you haven't audited your security, it's often safer to use the audited and secure OpenZeppelin library. Be careful, as people can make trivial mistakes!
Conclusion
What do you think about OpenZeppelin's Ownable?
If you want to operate your smart contract safely, utilizing libraries like this can help reduce stress and ensure a more secure blockchain experience. Give it a try!